1. Introduction to Timers in STM32F407
Timers are the heartbeat of microcontrollers, enabling them to handle time-based tasks with precision. Whether it's generating delays, producing PWM signals, counting events, or measuring intervals, timers play a crucial role in the smooth operation of embedded systems. Without them, achieving accurate time management would be a challenge, leading to inefficient and unreliable systems.
The STM32F407 microcontroller comes equipped with a variety of timers, each designed for specific tasks:
Basic Timers (TIM6, TIM7): These are straightforward 16-bit timers, primarily used for generating time delays or triggering events.
General-Purpose Timers (TIM2 to TIM5, TIM9 to TIM14): These versatile timers can handle a wide range of applications, from PWM generation to input capture and output compare. 16-bit (TIM3 and TIM4) or 32-bit (TIM2 and TIM5) up, down, up/down auto-reload counter.
Advanced-Control Timers (TIM1, TIM8): These timers are more sophisticated, featuring advanced options like dead-time insertion, making them ideal for motor control and other complex tasks.
2. Exploring the Features of Timer 2
General-Purpose Timer with Advanced Capabilities : Timer 2 is one of the general-purpose timers in the STM32F407, but don't let the term "general-purpose" fool you. This 32-bit timer can count from 0 to 4,294,967,295, offering a much larger range than the 16-bit timers.
Up to four independent channels for:
– Input capture
– Output compare
– PWM generation (Edge- and Center-aligned modes)
– One-pulse mode output
Clock Source and Flexibility : Timer 2 is driven by the APB1 bus clock, which typically runs at 42 MHz in the STM32F407. However, if the APB1 prescaler is set to 1 (meaning no division), Timer 2 can operate at an impressive 84 MHz. This flexibility is crucial, as the timer clock frequency directly influences the timer's counting speed.
16/32-bit Mode : One of the strengths of Timer 2 is its ability to operate in both 16-bit and 32-bit modes. Depending on your application’s needs, you can choose the mode that offers the best balance between range and granularity. While 16-bit mode allows counts up to 65,535, 32-bit mode extends this significantly, offering more precision or longer periods before overflow.
Multiple Channels for Versatile Applications : Timer 2 features four independent channels, each capable of different tasks:
Input Capture: Capturing the time of an external event.
Output Compare: Generating an interrupt or an output signal when the counter matches a specific value.
PWM Generation: Producing PWM signals for motor control, LED dimming, and more.(Edge- and Center-aligned modes)
One-pulse mode output: One-Pulse Mode (OPM) in the STM32F407 timer generates a single pulse in response to a trigger event. Unlike continuous PWM signals, OPM is used for precise, one-time pulse generation, making it ideal for tasks that require accurate, single-pulse output.
3. Block Diagram
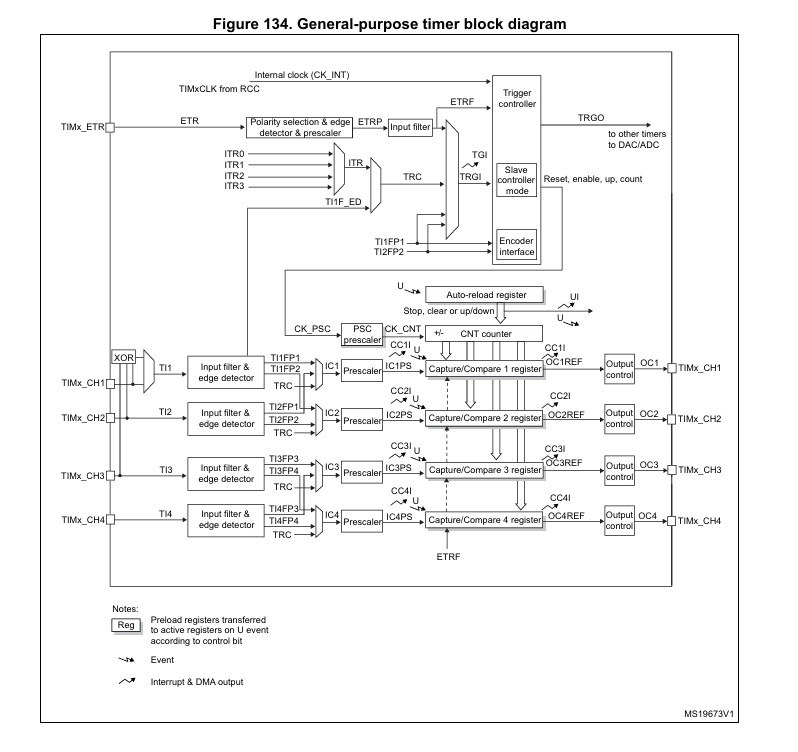
4. Configuring Timer 2 for a 1-Second Delay on the STM32F407 and to toggle the inbuild LED every 1 second
To set up Timer 2 on the STM32F407 to toggle led every 1 second, follow these steps:
Steps:
i. Enable the clock for the timer.
Enable the clock for Timer 2 by setting the appropriate bit in the RCC APB1ENR register.
ii. Enable clock for the GPIOD.
Since the onboard LED is connected to PD12, enable the clock for GPIOD by setting the relevant bit in the RCC AHB1ENR register.
iii. Configure PD12 as output.
Set PD12 as a general-purpose output in the GPIOD configuration register.
iv. Set up Timer 2.
Configure the timer prescaler to adjust the clock frequency to achieve a 1-second interval.
Set the auto-reload register (ARR) to determine the period (1 second) for the timer.
v. Configure the Interrupt (Optional)
Configue NVIC and update interrupt.
vi. Start Timer 2.
Enable the timer to begin counting.
vii. Toggle LED in the Timer 2 interrupt service routine (if using interrupts).
In the Timer 2 ISR, toggle the state of PD12 to make the LED blink.
Here’s a detailed description of the process:
i. Enable the clock for the timer
By Default STM32F407 uses the internal RC oscillator of 16MHz. Before you can use Timer 2, you need to enable its clock. This is done through the RCC (Reset and Clock Control) register, which controls the clocking of peripherals. By setting the bit 0 in the RCC_APB1ENR register, you activate the clock for Timer 2, allowing it to function.By default the clock frequency for timer will be 16MHz.
RCC->APB1ENR |= RCC_APB1ENR_TIM2EN;
ii. Enable clock for the GPIOD
Set the GPIODEN bit (3rd bit) in the RCC AHB1ENR register.
// RCC_AHB1ENR_GPIODEN is defined as (1 << 3)
RCC->AHB1ENR |= RCC_AHB1ENR_GPIODEN;
iii. Configure PD12 as output
Select Output Mode in GPIO Mode Register (MODER):
Set the MODER12[1:0] bits to 01 to configure PD12 as a general-purpose output.
// Configure PD12 as output
GPIOD->MODER &= ~(GPIO_MODER_MODER12_Msk); // Clear MODER12[1:0] bits
GPIOD->MODER |= (GPIO_MODER_MODER12_0); // Set PD12 as output (01)
iv. Set up Timer 2.
Prescaler (PSC) Register: The prescaler divides the timer clock to adjust the counting speed. To generate a 1-second delay, set the prescaler as follows:
Effective Timer Clock= Original Clock Frequency / (PSC+1)
Effective Timer Clock= 16MHz / (16000 - 1)
TIM2->PSC = 16000 - 1;
Whatever we write to the Prescaler (PSC) register, a 1 will be added to it.So to get 16000, we will subtract 1. If we input precaler value as 0, the actual value will be 1 since the controller automatically adds 1 to the given value by the user.So value of 1 means there is no division of the clcok and the actual frequency will be fed to the timer. (Detailed calculation is given below.)
Auto-Reload Register (ARR): The ARR register defines the timer's period. When the counter hits the value in ARR, the timer overflows, triggering an update event. For instance, with a PSC value that results in a 1 Hz timer clock, setting ARR to 1 will cause the timer to overflow every second.
// Set auto-reload register to 1000
TIM2->ARR = 1000 - 1;
ARR value should be - "Desired value - 1" since ARR starts counting from 0.Counting from 0 to 999 gives 1000 clock cycles. (Detailed calculation is given below.)
v. Configure the Interrupt (Optional)
If you want Timer 2 to generate an interrupt after each 1-second delay:
// Enable update interrupt
TIM2->DIER |= TIM_DIER_UIE;
// Configure NVIC for Timer 2 interrupt
NVIC_EnableIRQ(TIM2_IRQn);
vi. Start Timer 2.
Start Timer 2 by setting the CEN (Counter Enable) bit in the CR1 register:
// Enable Timer 2
TIM2->CR1 |= TIM_CR1_CEN;
vii. Toggle LED in the Timer 2 interrupt service routine (if using interrupts).
Interrupt Service Routine (ISR) (Optional) : Implement the ISR to handle the timer interrupt if enabled:
void TIM2_IRQHandler(void) {
if (TIM2->SR & TIM_SR_UIF) { // Check if update interrupt flag is set
TIM2->SR &= ~TIM_SR_UIF; // Clear the update interrupt flag
GPIOD->ODR ^= GPIO_ODR_OD12; // Toggle PD12 (connected to LED)
}
}
Counter Register (CNT): The CNT register holds the current value of the timer's counter. This value increments (or decrements in down-counting mode) based on the timer clock. You can read this register to check the current count or write to it to set a specific starting point.
Control Registers (CR1, CR2): The CR1 and CR2 registers control how the timer behaves. CR1 includes options for enabling the timer, setting the counting direction (up or down), and configuring the one-pulse mode. CR2 adds further configuration options, such as setting up master/slave mode for synchronizing Timer 2 with other timers.
5. Calculating Timer Settings for a 1-Second Delay
Let's say we want to generate a 1 second delay, the clcok frequency of the timer is 16MHz. Using a prescalar of 16000 will give 1000Hz which is 1KHz of clock frequency for the timer. Time period of 1KHz is 1millisecond which is .001 second.
Which means that the timer will count every 1 millisecond.
So inorder to generate a 1 second delay timer will have to count 1000 times since 1000 millisecond is 1 second.
Timer clock frequency = 16MHz/16000 - 1 = 1KHz
Time period = 1/f = 1/1KHz = 1millisecond
1000 * 1millisecond = 1000 millisecond = 1 second
Therefore ARR = 1000 - 1
6. Source code
#include "stm32f4xx.h"
// Function prototypes
void SystemClock_Config(void);
void GPIO_Init(void);
void Timer2_Init(void);
void TIM2_IRQHandler(void);
int main(void) {
// Initialize GPIOD for PD12
GPIO_Init();
// Initialize Timer2
Timer2_Init();
// Start Timer2
TIM2->CR1 |= TIM_CR1_CEN;
while (1) {
// The main loop can remain empty, everything is handled by the ISR
}
}
// GPIO Initialization for PD12
void GPIO_Init(void) {
// Enable GPIOD clock (PD is part of GPIOG)
RCC->AHB1ENR |= RCC_AHB1ENR_GPIODEN;
// Configure PD12 as output
GPIOD->MODER &= ~(GPIO_MODER_MODE12); // Clear mode bits
GPIOD->MODER |= (GPIO_MODER_MODE12_0); // Set mode to output
}
// Timer2 Initialization
void Timer2_Init(void) {
// Enable Timer2 clock
RCC->APB1ENR |= RCC_APB1ENR_TIM2EN;
// Set Timer2 prescaler and auto-reload register
TIM2->PSC = 16000 - 1; // Prescaler value (for 1kHz with 16MHz clock)
TIM2->ARR = 1000 - 1; // Auto-reload value (1 second interval at 1kHz)
// Enable Timer2 interrupt
TIM2->DIER |= TIM_DIER_UIE;
// Set Timer2 priority and enable IRQ
NVIC_SetPriority(TIM2_IRQn, 1);
NVIC_EnableIRQ(TIM2_IRQn);
}
// Timer2 Interrupt Handler
void TIM2_IRQHandler(void) {
// Check if update interrupt flag is set
if (TIM2->SR & TIM_SR_UIF) {
// Clear the update interrupt flag
TIM2->SR &= ~TIM_SR_UIF;
// Toggle PD12
GPIOD->ODR ^= GPIO_ODR_OD12;
}
}
7.OUTPUT
The output observed on a logic analyzer shows that the ON/OFF cycle (half the cycle) has a duration of 992 ms, which is approximately 1 second. The total time period is 1.98 seconds, which is approximately 2 seconds.
LED Blinking on STM32F407 Discovery board.
8. Practical Applications of Timer 2
Delay Generation : One of the simplest yet most essential uses of Timer 2 is generating delays. By carefully configuring the prescaler and ARR registers, you can create precise delays, perfect for tasks like blinking LEDs, generating timeouts, or pacing other processes.
PWM Generation : Timer 2 shines in PWM generation. By setting one of its channels to PWM mode, you can control the duty cycle of the output signal, which is invaluable for motor control, adjusting LED brightness, and similar applications.
Event Counting : Another powerful use of Timer 2 is counting external events. In input capture mode, the timer can count external pulses, making it ideal for frequency measurement or event counting.
Time Measurement : Timer 2 can also measure the time between two events. Using input capture mode, you can record the timer’s value at two points and calculate the time elapsed, which is useful in applications like pulse width measurement or speed sensing.
Comments